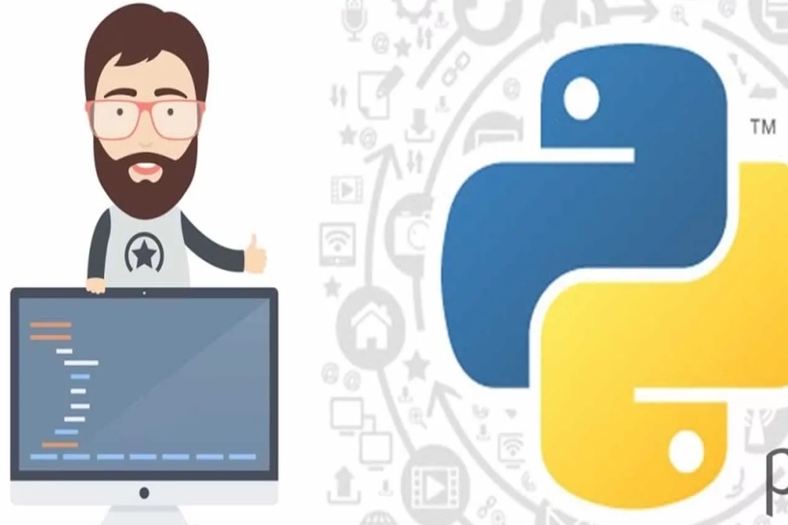
About Course
This is the most complete and at the same time simple course on the Python programming language!
If you’ve never programmed before, or you already know the basic syntax, or you need advanced Python topics, this course is for you! With over 100 lectures and over 14 hours of videos, this comprehensive course covers every possible aspect of the Python language!
This course contains quizzes, tests and homework assignments, as well as 3 big projects to build your portfolio of projects in Python!
In this course you will learn the Python language in practice – each lecture contains not only video lessons, but also the corresponding code! We’ll start by installing Python on your computer, there are instructions for different operating systems – Windows, MacOS and Linux !
What Will You Learn?
- Learn basic Python 3 syntax and data structures!
- Learn advanced Python features like the "collections" module and working with timestamps!
- Learn to use Object Oriented Programming!
- Explore advanced topics such as decorators and generators.
- Learn to create Jupyter Notebooks and .py files
- Learn to create GUIs in Jupyter Notebooks!
- Gain a confident understanding of Python language fundamentals!
Course Content
Course Overview
-
Introduction – Course Overview
-
Course Content Overview
-
Python 2 and Python 3. Python or Python?
-
FAQ
Installing Python
-
Command Line Basics
-
Installing Python
-
Running Python Code
Objects and Data Structures in Python
-
A reminder of where you can download Jupyter Notebooks.
-
Introduction to Python Data Types
-
Numbers
-
Numbers – Frequently Asked Questions
-
Test Questions on Numbers
-
Assigning Variables
-
Introduction to Strings
-
Indexing and Row Slicing
-
String Properties and Methods
-
Strings – Frequently Asked Questions
-
Test Questions by Line
-
Formatting Output for Strings
-
Output Formatting – Frequently Asked Questions
-
Lists in Python
-
Lists – Frequently Asked Questions
-
Checklist Questions
-
Dictionaries in Python
-
Dictionaries – Frequently Asked Questions
-
Dictionary Test Questions
-
Tuples in Python
-
Test Questions on Tuples
-
Sets in Python
-
Boolean values in Python
-
Test Questions – Sets and Boolean Values
-
Input/output (I/O) for files in Python
-
Resources for Additional Practice
-
Python Objects and Data Structures Test Review
-
Python Objects and Data Structures Test Solutions
Comparison Operators in Python
-
Comparison Operators in Python
-
Concatenating Comparison Operators Using Logical Operators
-
Test Questions on Comparison Operators
Python Operators
-
If, Elif and Else Statements in Python
-
For Loops in Python
-
While Loops in Python
-
Useful Operators in Python
-
List Comprehensions in Python
-
Python Operators Test Review
-
Python Operator Test Solutions
Methods and Functions
-
Python Methods and Documentation
-
Functions in Python
-
*args and **kwargs in Python
-
Function Exercises
-
Review of Function Exercises
-
Exercise Solutions – Level Warm Up
-
Exercise Solutions – Level 1
-
Exercise Solutions – Level 2
-
Solutions to Exercises – Complex Problems
-
Lambda expressions, Map and Filter functions
-
Nested Operators and Scopes
-
Functions and Methods – Homework
-
Helpful Tips for Functions and Methods Assignment
-
Homework Solutions for Functions and Methods
Project – 1
-
Overview of the First Python Project
-
Project Help
-
Project Solution Review 1 – Part 1
-
Project Solution Review 1 – Part 2
Object Oriented Programming (OOP)
-
OOP: Introduction
-
OOP: Attributes and the Class Keyword
-
OOP: Classes, Objects, Attributes and Methods
-
OOP: Inheritance and Polymorphism
-
OOP: Special Methods (Magic/Dunder)
-
OOP: Homework
-
OOP: Homework Solution
-
OOP: Problem
-
OOP: Solution to a Problem
Modules and Packages
-
Pip Install and PyPi
-
Modules and Packages
-
__name__ and “__main__”
Errors and Exception Handling
-
Errors and Exception Handling
-
Homework – Errors and Exception Handling
-
Homework Solutions – Errors and Exception Handling
-
Pylint Review
-
Running tests using the Unittest library
Project – 2
-
Project 2 Overview
-
Solution – Card and Deck classes
-
Solution – Hand and Chip classes
-
Solution – functions for Game Play
-
Solution – Final Gameplay script
Decorators in Python
-
Overview of Decorators in Python
-
Decorators – Homework
Python Generators
-
Generators in Python
-
Generator Homework Review
-
Generator Homework Solutions
Final Project
-
Final Project
Advanced Python Modules
-
Collections module – counter
-
Collections module – defaultdict
-
Collections – OrderedDict module
-
Collections module – namedtuple
-
Datetime
-
Python Debugger – pdb
-
Measuring code time – timeit
-
Regular Expressions – re
-
StringIO
Advanced Python Objects and Data Structures
-
Advanced Numbers
-
Advanced Strings
-
Advanced Sets
-
Advanced Dictionaries
-
Advanced Lists
-
Advanced Python Objects Test
-
Solutions for the Advanced Python Objects Test
Additional Materials – Introduction to Graphical Interfaces (GUI)
-
Introduction to Graphical Interfaces (GUIs)
-
A note about ipywidgets
-
Interactivity with Graphical Interfaces (GUIs)
-
Basics of Working with Widgets
-
List of Available Widgets
-
Styling and Widget Layouts
-
Example of Using Widgets