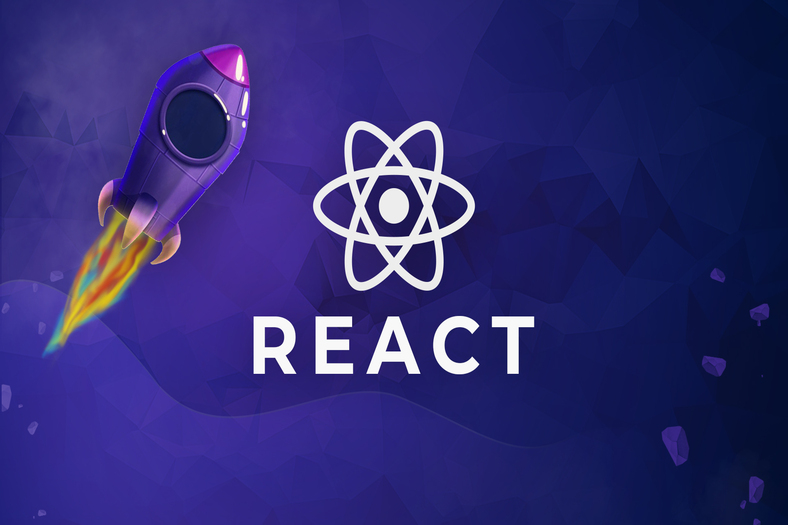
About Course
Learn app development with the most popular JS library in a simple and understandable language for beginners! React.js is currently the most popular JavaScript library that you can use on the front end to create modern user interfaces.
In this course, you will learn modern React from the very beginning, step by step, using practical and interesting code examples, important theory about how React works under the hood, as well as many practical tasks. All you need is basic knowledge of web development and JavaScript.
You will learn React in practice, using all modern patterns, understand key concepts, as well as advanced concepts and related topics. You will not only learn React, but also learn how to solve problems, learn how to structure and organize code. All this will help you become a React developer!
What Will You Learn?
- How React works
- Creating React Components
- Creating user interfaces
- What are props and two-way binding
- Events and state for creating interactive applications
- How React works under the hood
- Working with lists and content based on conditions
- Ways to style components
- Hooks
- Using built-in hooks and creating custom hooks
- Debugging React applications
- Styling with "styled components" & CSS modules
- Working with fragments and portals
- Dealing with Side Effects
- Class-Based Components
- Working with Http requests
- Managing data entry from forms
- Redux - Fundamentals + Advanced
- React Router
Course Content
Introduction
-
Introduction
-
What is React.js?
-
Why Use React?
-
Creating Single Page Applications (SPAs) with React
-
React Alternatives (Angular, Vue)
-
Course Overview
-
Setting up Development Tools
React Basics
-
Application Code “Expense Accounting”
-
Section Brief Overview
-
Components – Fundamental Blocks of React Applications
-
Declarative Programming
-
Creating a Project
-
Project preparation
-
React Project Overview
-
Introducing JSX
-
How React Works
-
Creating a Component
-
More Complex JSX Code
-
Adding CSS Styles
-
Displaying Dynamic Data in JSX
-
Transferring Data Using Props
-
JavaScript Logic in Components
-
Composition (props.children)
-
First Results
-
More about JSX
-
Organizing Component Files
-
Alternative Function Syntax
State and Working with Events
-
Section Brief Overview
-
“Listening” to Events and Working with Event Handlers
-
How Functions-Components Are Performed
-
Working with “State”
-
More about the “useState” Hook”
-
Adding an Input Form
-
Listening to User Input
-
Working with Multiple States
-
Using One State
-
Updating a State Dependent on a Previous State
-
Processing Form Submissions
-
Adding Two-Way Linking
-
Communication of Components Heir-Parent (Bottom-Up)
-
Components – Controlled vs Uncontrolled, with State vs without State
Displaying Content by Condition and Lists
-
Section Brief Overview
-
Displaying Data Lists
-
Using Lists with State
-
Keys
-
Displaying Content by Condition
-
Adding an Expense Chart
-
Dynamic Styles
-
Completing Application Development
Styling Components
-
Section Brief Overview
-
Dynamically Adding Inline Styles
-
Dynamically Adding CSS Classes
-
Introduction to Styled Components
-
Styled Components and Dynamic Props
-
Styled Components and Media Queries
-
Using CSS Modules
-
Dynamic Styles and CSS Modules
-
Code for the entire project
Debugging React Applications
-
Section Brief Overview
-
Error Messages in React
-
Code and Warning Analysis
-
Working with Breakpoints
-
Using React DevTools
-
Code for the entire project
Independent Work – Challenge No. 1
-
Section Brief Overview
-
Adding a CreateUser Component
-
Adding a Card Component
-
Adding a Button Component
-
Data Input State Management
-
Adding Validation and Reset Logic
-
Adding a UserList Component
-
Managing Your User List Through Status
-
Adding an ErrorModal Component
-
Error Status Management
-
Code for the entire project
Fragments, Portals, Refs
-
Section Brief Overview
-
JSX Limitations
-
Creating a Wrapper Component. Fragments
-
Portals
-
Working with Portals
-
Working with refs
-
Code for the entire project
React Advanced – Side Effects, Reducers, Context
-
Section Brief Overview
-
What are Side Effects
-
Using the useEffect Hook
-
useEffect and Dependencies
-
Using the Cleaning Function
-
Reducers
-
Using the useReducer Hook
-
useReducer and useEffect
-
useState vs useReducer
-
Context
-
Using the Context API
-
Using the useContext Hook
-
Dynamic Context
-
Custom Context Provider Component
-
Limitations Context
-
Hook Rules
-
Refactoring the Input Component
-
forwardRef & useImperativeHandle
-
Code for the entire project
Japan Cuisine – Food Ordering App
-
Section Brief Overview
-
Creating a Project Structure
-
Adding a Header Component
-
Adding a HeaderCartButton Component
-
Adding Components to Display Dishes
-
Dishes Display
-
Adding a Form
-
Minor Bug Fix
-
Adding a Cart Component
-
Adding a Modal Window
-
Cart and Modal State Management
-
Adding Cart Context
-
Using Context
-
Adding Reducer to Cart
-
Using refs
-
Displaying Cart Items
-
Complication of Logic Reducer
-
Adding Element Removal Functionality
-
Using useEffect
-
Code for the entire project