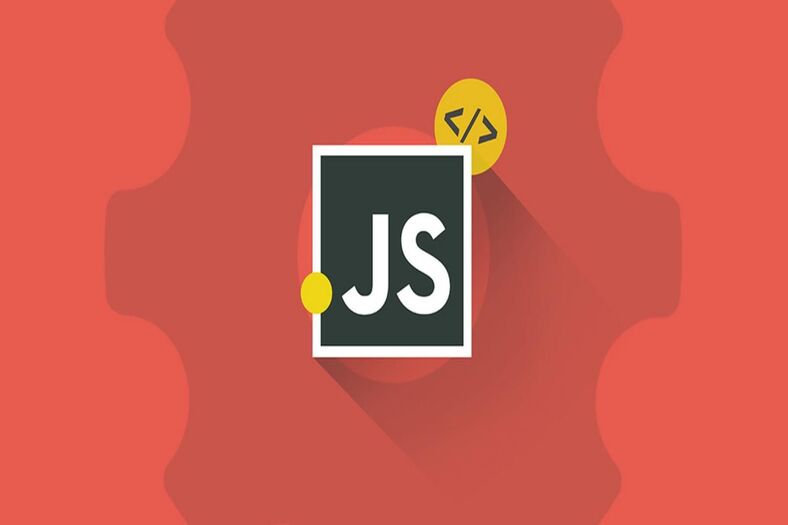
About Course
JavaScript is THE most important programming language you need to learn as a web developer, and by taking this course you won’t miss a single thing you need to know as a JavaScript developer!
Why is this JavaScript course right for you? This is the newest and most comprehensive JavaScript course that will take you from JavaScript basics to advanced levels to ace your interview and become a professional developer.
You’ll learn modern JavaScript from the very beginning, step-by-step, with practical and interesting code examples, important theory about how JavaScript works under the hood, and plenty of hands-on assignments.
This course contains topics for beginners, intermediate and even advanced levels. Not only will you learn the JavaScript language, but you will also learn how to solve problems, learn how to structure and organize code.
What Will You Learn?
- Become an advanced, confident and modern JavaScript developer from scratch
- Learn the basics of JavaScript and any programming language: variables, logic, conditionals, loops, functions, arrays, objects, etc.
- Be ready to go by understanding how JavaScript actually works under the hood
- Learn to think and work like a developer: problem solving, research, workflows
- You will practice a lot
- Learn to debugging your code
- Learn to style web pages using JavaScript
- Learn the basics of HTML & CSS
- Learn to manipulate DOM elements using JavaScript
- Create 5 beautiful projects for your portfolio
- Learn how to think and work like a developer: problem solving, research, workflows
- Learn the basics of modern React
- And much more!
Course Content
Introduction
-
Introduction
-
IMPORTANT! FAQ – Frequently Asked Questions
-
Notes on assignments
JavaScript Basics
-
What is JavaScript?
-
Tools
-
Installing VS Code. Windows
-
Installing VS Code. Mac
-
Ways to Use JavaScript
-
Console
-
Variables. Comments
-
let and const
-
Data types
-
Type Conversion
-
Operations with numbers
-
Strings
-
Template strings
-
Task “Questionnaire”
-
Comparison Operators
-
Operator precedence
-
Abbreviated form of recording operators
-
Task “Excess weight”
-
If Else Statement
-
Truthy and Falsy meanings
-
Boolean logic
-
Switch Statement
-
Statements & Expressions
-
JavaScript versions
-
Strict Mode
-
Functions
-
Arrays
-
Array Methods
-
Lesson draft
00:00 -
Objects. Dot vs Bracket Notation
-
Object Methods
-
For loop
-
Loops and Arrays
-
Break & Continue
-
Iterate in reverse order. Loop within a Loop
-
While loop
Required Developer Skills
-
Code for the entire section
-
Setting up VS Code
-
Setting up Development Environment
-
How to Learn Programming
-
Think Like a Programmer
-
Searching for information on the Internet
-
Debugging
-
Debugging code using the console and breakpoints
Crash Course HTML & CSS
-
Code for the entire section
-
Web page structure. Basic html elements
-
Attributes, class, id
-
Styling with CSS
-
CSS Box Model
DOM Basics
-
Code for the entire section
-
Project No. 1. Guess the Number!
-
What is DOM?
-
Element Manipulation
-
Events & Event Handlers
-
Implementation of logic
-
Modifying CSS Using JavaScript
-
Challenge – start the game over
-
Best result
-
Code refactoring
How JavaScript Works – Looking Under the Hood
-
Code for the entire section
-
JavaScript Overview
-
JavaScript engine
-
Execution Context & Call Stack
-
Scope & Scope Chain
-
Scope in practice
-
Variable Environment. Hoisting & TDZ
-
Variable Environment. Hoisting & TDZ in practice
-
Keyword this
Data Structures And Strings In Modern JavaScript
-
Code for the entire section
-
Destructuring Arrays
-
Destructuring Objects
-
Spread operator
-
Rest Pattern and Rest Parameters
-
Calculation of Logical Expressions Using a Short-Circuit Evaluation
-
Nullish Coalescing – Null Coalescing Operator
-
Loops and Arrays. Loop for
-
Improved Object Literals
-
Optional Chaining
-
Loops and Objects
-
Set
-
Which Data Structure Should You Use?
More About Features
-
Code for the entire section
-
Default Options
-
Passing Arguments. Values vs Links
-
First Class Functions vs Higher Order Functions
-
Functions Receiving Callback Functions
-
Functions Returning Functions
-
Call() and apply() methods
-
Bind() method
-
Immediately Invoked Function Expression (IIFE)
-
Closures
-
More Examples About Closures
Working With Arrays
-
Code for the entire section
-
Simple Array Methods
-
Iterating Arrays Using a forEach Loop
-
forEach With Map And Set
-
Project No. 4. Application “Simply Bank”
-
Creating DOM Elements
-
Data Transformation: map(), filter(), reduce()
-
Map() method
-
Nicknames calculation
-
Filter() method
-
Reduce() method
-
Displaying the balance in the application
-
Method Chaining
-
Method Chaining In Application
-
Find() method
-
Implementation of Login
-
Implementation of Transfers
-
FindIndex() method
-
Some() and every() methods
-
Loan Request
-
Flat() and flatMap() methods
-
Sorting Arrays
-
Sorting Transactions in the Application
-
Other Ways to Create and Fill Arrays
-
Array.from() in a web application
-
Which Method Should I Use?
-
Array Methods in Practice
Numbers, Dates, Timers
-
Code for the entire section
-
Converting And Validating Numbers
-
Working with Math
-
We make changes to the Application
-
Operator Remainder
-
Using the Remainder Operator in the Application
-
BigInt
-
Working with Dates
-
Adding Dates to the Application
-
Internationalization of Dates
-
Internationalization of Numbers
-
Internationalizing Numbers in an Application
-
Timers
-
Implementation of Countdown