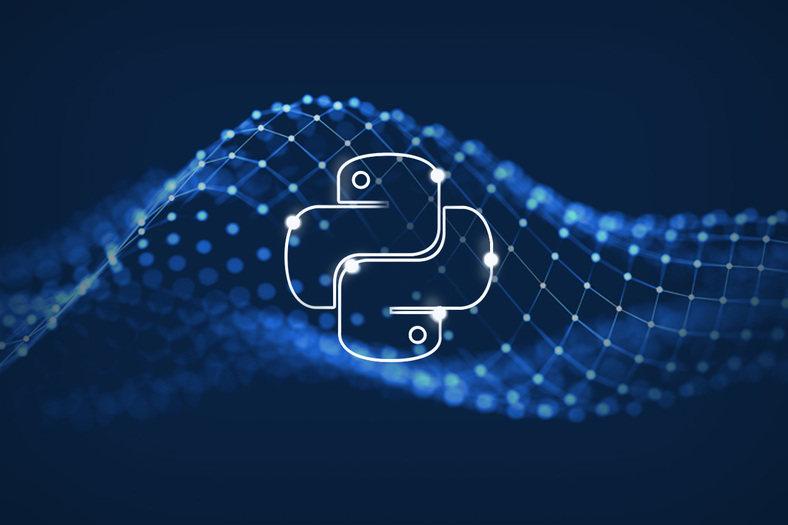
About Course
Become a Python programmer and learn one of the most in-demand skills in modern development!
This is the most comprehensive yet simple course on the Python programming language! Whether you’ve never programmed before, whether you already know the basic syntax, or if you want to learn about Python’s advanced features, this course is for you!
In this course, we will learn programming in Python 3. This course is designed for beginners who have never programmed before, as well as existing programmers who want to expand their career opportunities by learning Python. The fact is that Python is one of the most popular programming languages in the world – huge companies like Google use it in mission-critical applications like Google Search.
Python is the number one language for machine learning, data science, and artificial intelligence. To get that high-paying job, you need expert knowledge of Python, and that’s what you’ll get from this course.
What Will You Learn?
- Gain a fundamental understanding of the Python programming language
- Get Python Object Oriented Programming (OOP) Skills on Your Resume
- Understand complex topics like decorators, timestamp, etc.
- Acquire the necessary Python skills to move into specific industries - machine learning, data science, etc.
- Data types
- Indexing & Slicing
- Formatting strings
- Lists, Dictionaries, Tuples, Sets
- List, Dictionary and Set Comprehension
- Nested Loops
- *args. **kwargs.
- Lambda expressions
- Multiple inheritance
- Method Resolution Order (MRO)
- Modules and packages
- File I/O
- @wraps
- Testing
- Test Driven Development (TDD)
- HTTP & API
Course Content
Introduction
-
Introduction
-
IMPORTANT! FAQ – Frequently Asked Questions
-
Notes on assignments
Installation of tools. Windows
-
Installing Python
-
Assignment for the lecture “Installing IntelliJ IDEA” for Windows
-
Installing IntelliJ IDEA
Python Basics
-
Hello world!
-
Displaying text on screen
-
Data types
-
Numbers. int & float
-
Evaluating Arithmetic Expressions
-
Variables
-
Creating Variables
-
Strings
-
Working with Strings
-
Lines. Indexing & Slicing
-
Indexing & Slicing
-
String properties. Methods
-
String properties. Methods
-
Formatting strings
-
Formatting strings
-
Lists
-
Lists
-
Dictionaries
-
Dictionaries
-
Tuples
-
Tuples
-
Sets
-
Sets
-
Notes on the lecture “Booleans. Comparison Operators”
-
Booleans. Comparison Operators
-
Booleans. Comparison Operators
-
Logical operators
-
Conditional statement if elif else
-
Conditional statement if elif else
-
For loop
-
For loop
-
While loop
-
Some frequently used functions and operators
-
List Comprehension
-
List Comprehension
-
Dictionary and Set Comprehension
-
Nested Loops
-
Nested Lists
Functions
-
Functions. Introduction
-
Creating Functions
-
Creating Functions
-
*args. **kwargs.
-
*args. **kwargs.
-
Lambda expressions
-
Variable Scope
Object-oriented programming (OOP)
-
Object-oriented programming (OOP). Introduction
-
Attributes
-
Attributes
-
Methods
-
Methods
-
Class Methods
-
Inheritance. Polymorphism
-
Inheritance. Polymorphism
-
Multiple inheritance
-
Method Resolution Order (MRO)
-
Special (magic) methods
-
Special (magic) methods
Modules and packages
-
Built-in modules
-
Built-in modules
-
Creating your own modules
-
Creating your own modules
-
External modules
-
__name__ and ‘__main__’
File I/O
-
Reading text files
-
Writing text files
-
Binary number system
-
Writing binary files
-
Pickle module
-
Shelve module
-
Working with data using the shelve module
-
Updating data using the shelve module
-
Converting a dictionary to a shelve object
Error processing
-
Types of errors
-
Calling errors
-
try except
-
else finally
Iterators and generators
-
Iterable & iterator
-
Custom iterable
-
Generator functions
-
Day of the week generator
-
Even-odd
-
Infinite Generators
-
Infinite number square generator
-
Generator expressions